Your content goes here. Edit or remove this text inline or in the module Content settings.
You can also style every aspect of this content in the module Design settings and even apply custom CSS to this text in the module Advanced settings.
Your content goes here. Edit or remove this text inline or in the module Content settings.
You can also style every aspect of this content in the module Design settings and even apply custom CSS to this text in the module Advanced settings.
Your content goes here. Edit or remove this text inline or in the module Content settings.
You can also style every aspect of this content in the module Design settings and even apply custom CSS to this text in the module Advanced settings.
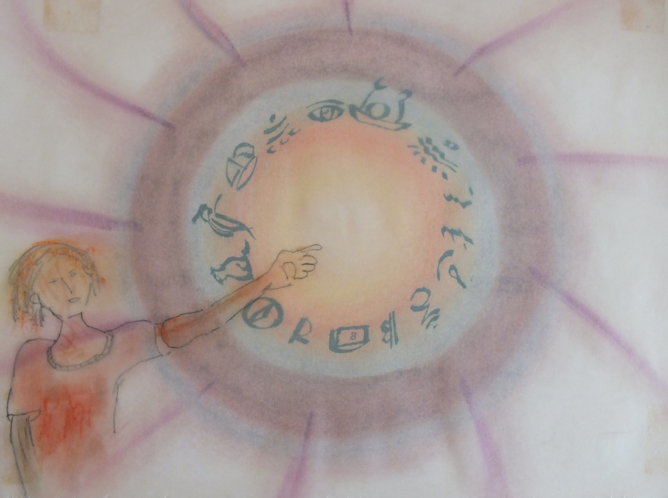
def fib(n):
a, b = 0, 1
while a < n:
from machine import Pin, I2C
from font8x8 import font8x8 # Importer les polices externes
# Initialisation I2C
i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=400000)
oled_addr = 0x3C # Adresse de l'OLED
# Création du buffer pour stocker l'affichage (1024 octets)
buffer = bytearray(1024)
# Fonction pour envoyer une commande à l'écran
def oled_cmd(cmd):
i2c.writeto(oled_addr, b'\x00' + bytes([cmd]))
# Fonction pour dessiner un élément 8x8 pixels dans le buffer
def draw_8x8(x, y, sprite):
page = y // 8
for col in range(8):
if 0 <= x + col < 128:
buffer[(page * 128) + (x + col)] = sprite[col]
# Fonction pour dessiner un caractère en 8x8
def draw_char(x, y, char):
if char in font8x8:
draw_8x8(x, y, font8x8[char])
# Fonction pour afficher du texte
def draw_text(x, y, text):
for i, char in enumerate(text):
draw_char(x + i * 8, y, char)
update_oled()
# Fonction pour mettre à jour l'écran
def update_oled():
for page in range(8):
oled_cmd(0xB0 + page)
oled_cmd(0x00)
oled_cmd(0x10)
i2c.writeto(oled_addr, b'\x40' + buffer[page * 128:(page + 1) * 128])
# Effacer l'écran avant l'affichage
buffer[:] = b'\x00' * 1024
update_oled()
# Afficher "ABC" en haut à gauche
#draw_text(0, 0, "ABCDEFGHIJKLMNOP")
#draw_text(0, 8, "QRSTUVWXYZabcdef")
#draw_text(0, 16,"ghijklmnopqrstuv")
#draw_text(0, 24,"wxyz !'#$%&()*+,")
draw_text(12, 12,"àäéöèü")
fib(1000)